A better approach to populating dropdown lists in webapps with schema enum values consists of pulling the values directly from the schema rather than hardcoding the values in the webapp front end as the downside is that a webapp will require updating if these values change or new ones are added.
Start by adding a JS activity and include the following function.
/* recipient status enum */
function fetchEnum(s,e) {
var schema = application.getSchema(s);
var enumeration = schema.enumerations.filter(function(enum) {return enum.label == e})[0];
var enumObj = []
for each(var s in enumeration.values) {
enumObj.push({"value":s.value,
"label":s.label
})
}
return enumObj
}
The next step is to call the function and configure the schema/enum names as such
var myEnum = JSON.stringify(fetchEnum("nms:yourSchema","yourEnum"));
In the following example, I have a custom status enum in the recipient's schema.
<enumeration basetype="byte" name="schStatus">
<value label="Subscriber" name="subscriber" value="1"/>
<value label="Lead" name="lead" value="2"/>
<value label="MQL" name="mql" value="3"/>
<value label="SQL" name="sql" value="4"/>
<value label="Opportunity" name="opportunity" value="5"/>
<value label="Client" name="client" value="6"/>
<value label="Other" name="other" value="0"/>
</enumeration>
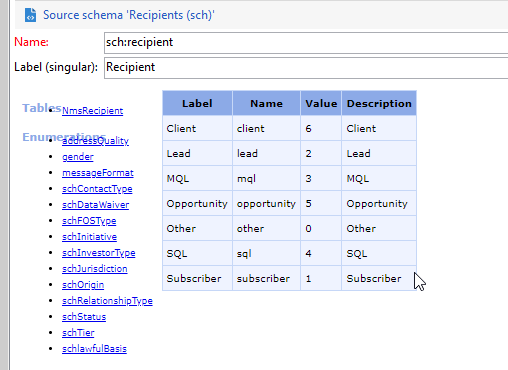
To retrieve all the values and labels; configure the function as following.
ctx.vars.statusJSON = JSON.stringify(fetchEnum("nms:recipient","schStatus"));
the values will be available through the ctx context which can be parsed and assigned to local variables using javascript.
<statusJSON>[{"value":"1","label":"Subscriber"},{"value":"2","label":"Lead"},{"value":"3","label":"MQL"},{"value":"4","label":"SQL"},{"value":"5","label":"Opportunity"},{"value":"6","label":"Client"},{"value":"0","label":"Other"}]</statusJSON>
Lets say your form contains the following code block which contains a dropdownlist element with empty option values.
<div class="form-floating mb-3">
<select name="status" class="form-select" id="status" aria-label="" placeholder="Choose">
<option></option>
</select>
<label for="status">Status</label>
</div>
Add the following code to your javascript, which references the ctx string and parses it as JSON then a function will retrieve key:value pairs and populate your dropdownlist element.
<script type="text/javascript">
jQuery(document).ready(function() { //on document ready
//retrieve status value string from ctx vars variable and parse as JSON
var status = JSON.parse(document.controller.getValue('/ctx/vars/statusJSON'));
//iterate array of objects and append to #status element values and text key values.
$.each(status, function(i, key) {
$('#status').append($('<option />', {
value: (key.value),
text: key.label
}))
});
</script>
});
Here is your final product.
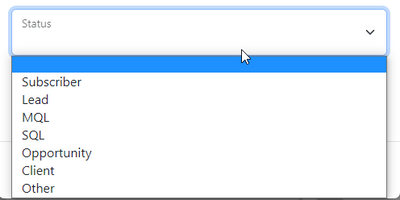
<div class="form-floating mb-3">
<select name="status" class="form-select" id="status" aria-label="" placeholder="Choose">
<option></option>
<option value="1">Subscriber</option>
<option value="2">Lead</option>
<option value="3">MQL</option>
<option value="4">SQL</option>
<option value="5">Opportunity</option>
<option value="6">Client</option>
<option value="0">Other</option>
</select>
<label for="status">Status</label>
</div>
Dependencies: jQuery
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.